TValue REST API Documentation
Welcome to the TValue REST API
This REST web service allows access to a subset of TValue 6 features as REST API endpoints. To access the TValue REST API and TValue .NET calculation engine, you'll need to obtain an access key.
Please contact us with any questions, comments, or feedback.
Introduction
TimeValue Software is the world leader in amortization, interest, and time value of money calculations. The TValue REST API allows you to power your desktop and web applications with the same world class TValue .NET calculation engine as our signature TValue 6 desktop application.
To make calculations using the TValue REST API, you will need to obtain a Customer ID. To obtain a customer ID, call us at 800-426-4741 to speak with one of our team members.
Once you have obtained a Customer ID, you can familiarize yourself with the capabilities of each endpoint using the TValue REST API Sandbox. You can then continue to the endpoint documentation below to learn how to call each calculation.
Endpoints
GetServerDateTime: To test your connection and ability to correctly call the web service, start by making a GET request to our testing endpoint, GetServerDateTime
const getServerDateTime = async(input) => {
const Url = "https://tvaluerestws.com/WebApi/GetServerDateTime/"
const Settings = {
method: 'GET',
headers: {
'Content-Type': 'application/json'
},
};
try{
const FetchResponse = await fetch(Url, Settings);
const Data = await FetchResponse.json();
console.log(Data);
} catch(e){
console.log(e);
}
}
You should receive a response that looks like the following that confirms your connection:
{"ServerDateTime":"2023-03-29 16:22:01:646"}
-
CustomerId:
Your unique customer ID
- CreateAmSchedule:
Boolean
- Rounding Type:
Accepted options are Ignore, LastPayment, FirstLoan, Balloon, SpecificLine, and OpenBalance
- SpecificLine:
OPTIONAL: Expects a number to reference for SpecificLine rounding.
- LineAdjustment:
OPTIONAL: Expects AllAmounts, FirstAmount, or LastAmount
- Document:
JSON Cash Flow Document. See Cash Flow Matrix for further details
const CustomerId = ''YOUR_API_KEY'';
const CreateAmSchedule = false;
const RoundingType = 'Ignore';
const SpecificLine = 2;
const LineAdjustment = "AllAmounts";
const CalculateDocument = {
"CFM" :
[
{
"Amount" : 10000000.0,
"Event" : "Loan",
"Number" : 1,
"StartDate" : "2021-01-01"
},
{
"Amount" : "Unknown",
"Event" : "Payment",
"Number" : 24,
"Period" : "Monthly",
"StartDate" : "2021-02-01"
}
],
"Label" : "",
"Period" : "Monthly",
"Rate" : 0.05,
"Schema" : 6,
"YearLength" : 365
};
const calculateObject = {
CustomerId,
CreateAmSchedule,
RoundingType,
SpecificLine,
LineAdjustment,
Document: CalculateDocument
}
const calculateUnknownJson = async(input) => {
const Url = "https://tvaluerestws.com/WebApi/calculate/"
const Settings = {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(input)
};
try{
const FetchResponse = await fetch(Url, Settings);
const Data = await FetchResponse.json();
console.log(Data);
} catch(e){
console.log(e);
}
}
const calculateJson = () => calculateUnknownJson(calculateObject);
calculateJson();
You should receive a response that looks like the following:
{
"CFM": {
"CFM" :
[
{
"Amount" : 10000000.0,
"Event" : "Loan",
"Number" : 1,
"StartDate" : "2021-01-01"
},
{
"Amount" : 438714.0,
"Event" : "Payment",
"Number" : 24,
"Period" : "Monthly",
"StartDate" : "2021-02-01"
}
],
"Label" : "",
"Period" : "Monthly",
"Rate" : 0.05,
"Schema" : 6,
"TValue Engine Build" : "Mar 8 2023 07:22:25",
"Unknowns" : { "Type" : "Amount", "Value" : 438714.0 },
"YearLength" : 365
}
}}
The TValue .NET Calculation Engine has solved for the dollar amount of each payment as noted both in the cash flow matrix and in the "Unknowns" key within the response.
Note that if you have marked CreateAmSchedule as True, you will receive an amortization table as JSON in addition to the Cash Flow Matrix.
- CustomerId:
Your unique customer ID
- BalanceDate:
YYYY-MM-DD format
- Document:
JSON Cash Flow Document. See Cash Flow Matrix for further details
const CustomerId = '';
const BalanceDate = "2022-01-02";
const BalanceDocument = {
"CFM" :
[
{
"Amount" : 10000000.0,
"Event" : "Loan",
"Number" : 1,
"StartDate" : "2021-01-01"
},
{
"Amount" : 438714.0,
"Event" : "Payment",
"Number" : 24,
"Period" : "Monthly",
"StartDate" : "2021-02-01"
}
],
"Label" : "",
"Period" : "Monthly",
"Rate" : 0.05,
"Schema" : 6,
"YearLength" : 365
}
const balanceObject = {
CustomerId,
BalanceDate,
Document: BalanceDocument
}
const getBalanceJson = async(input) => {
const Url = "https://tvaluerestws.com/WebApi/Balance/"
const Settings = {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(input)
};
try{
const FetchResponse = await fetch(Url, Settings);
const Data = await FetchResponse.json();
console.log(Data);
} catch(e){
console.log(e);
}
}
const balanceJson = () => getBalanceJson(balanceObject);
balanceJson();
Your response should include a Principal & Interest balance:
{ Principal: 5124714, Interest: 702 }
The TValue .NET Calculation Engine has calculated the principal and interest balances for the given date.
- CustomerId:
Your unique customer ID
- Rate:
Percent as a decimal. Ex: 0.05 for 5%
- RateType:
The input rate type. Accepted options are Nominal, Effective, Periodic, and Daily.
- CompoundingPeriod:
The compounding period for the calculation. Accepted options are Annual, Biweekly, Continuous, Daily, ExactDays, FourMonth, FourWeek, HalfMonth, Monthly, Quarterly, Semiannual, TwoMonth, and Weekly
- YearLength:
Length of a year for the purpose of calculations. 365, 360, and 364 are accepted options.
const CustomerId = ''YOUR_API_KEY'';
const Rate = 0.05;
const RateType = "Nominal";
const CompoundingPeriod = "Monthly";
const YearLength = 365;
const ratesObject = {
CustomerId,
Rate,
RateType,
CompoundingPeriod,
YearLength,
}
const getRatesJson = async(input) => {
const Url = "https://tvaluerestws.com/WebApi/Rates/"
const Settings = {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(input)
};
try{
const FetchResponse = await fetch(Url, Settings);
const Data = await FetchResponse.json();
console.log(Data);
} catch(e){
console.log(e);
}
}
const ratesJson = () => getRatesJson(ratesObject);
ratesJson();
Your response should look like the following
{
NominalRate: '0.05',
EffectiveRate: '0.051161897881732976',
PeriodicRate: '0.004166666666666667',
DailyRate: '0.00013698630136986303'
}
The rates you get back solve for the different interest rate types available from the TValue .NET Calculation engine.
Cash Flow Matrix
Aside from creating the proper connection with the TValue REST API and passing your API key, the most important part of getting correct calculations is formatting your Cash Flow Matrix (CFM) correctly. If you are familiar with the TValue Software, the format should be familiar to you, but if not, here's an explanation of the shape the API expects and what each key/value represents:
- Amount: The cash amount of the line item. NOTE: This should be in cents, not dollars.
- Event: Accepted options come in pairs: Loan and Payment or Deposit and Withdrawal
- Number: Count of consecutive identical events
- StartDate: The date of the first event in the series
- Period: The period with which the event repeats. Accepted options include:
Weekly, Biweekly, HalfMonth, Monthly, 2-Month, Quarterly, 4-Month, 4-Week, Semiannual, and Annual.
NOTE: Period cannot be more frequent than the compounding period, so not every option is acceptable for every compounding period.
Examples: See the following examples that might be used for calculating a balloon payment or solving for an unknown payment amount respectively:
const BalloonDocument = {
"CFM" :
[
{
"Amount" : 10000000.0,
"Event" : "Loan",
"Number" : 1,
"StartDate" : "2023-03-01"
},
{
"Amount" : 470735.0,
"Event" : "Payment",
"Number" : 24,
"Period" : "Monthly",
"StartDate" : "2023-04-01"
},
],
"Label" : "Rounding with Balloon",
"Period" : "Monthly",
"Rate" : 0.12,
"Schema" : 6,
"YearLength" : 365
}
const CalculateDocument = {
"CFM" :
[
{
"Amount" : 10000000.0,
"Event" : "Loan",
"Number" : 1,
"StartDate" : "2021-01-01"
},
{
"Amount" : "Unknown",
"Event" : "Payment",
"Number" : 24,
"Period" : "Monthly",
"StartDate" : "2021-02-01"
}
],
"Label" : "",
"Period" : "Monthly",
"Rate" : 0.05,
"Schema" : 6,
"YearLength" : 365
};
Frequently Asked Questions (FAQ)
Note: It is highly recommended that you become comfortable making the types of calculations you will be implementing with the TValue REST API with the TValue 6 Amortization Software desktop software first. Familiarity with TValue 6 Amortization Software will give you context as to how the TValue .NET calculation engine document is laid out and how the data and options from the document are processed when running calculations.
If you are able to properly make requests to the TValue REST API endpoints, but are having trouble making specific calculations, you can check out the TValue Glossary or contact TimeValue Support.
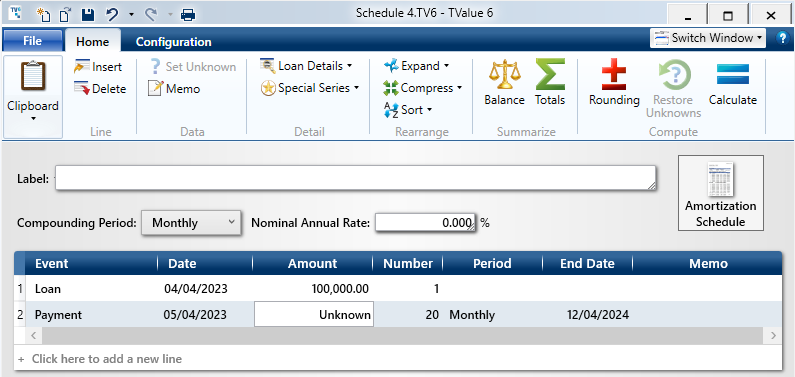
When you create your JSON CFM document, simply replace the value of the key you'd like to solve for to "unknown". See the below example that solves for an unknown payment amount for a set number of consecutive payments.
const CalculateDocument = {
"CFM" :
[
{
"Amount" : 10000000.0,
"Event" : "Loan",
"Number" : 1,
"StartDate" : "2021-01-01"
},
{
"Amount" : "Unknown",
"Event" : "Payment",
"Number" : 24,
"Period" : "Monthly",
"StartDate" : "2021-02-01"
}
],
"Label" : "",
"Period" : "Monthly",
"Rate" : 0.05,
"Schema" : 6,
"YearLength" : 365
};
In order to ensure accurate calculations, be sure to use cents as your unit for monetary amounts within your CFM documents.
We understand privacy is important in financial calculations. We log basic information each time you hit an endpoint, but we do not save the CFM document requests or responses.